Public controllers
- Home
- Developing modules
- Public controllers
Any controllers which don't implement ManagedAdminController
are considered 'public' or 'front-end' controllers - whether or not they are actually publically accessible or have authentication controls.
These are created simply by extending the Controller
class and adding public methods, then registering routes to call the methods.
Here's the most basic kind of public controller, which simply sets the title of the page to be "Hello World!" and displays it in a heading. It does this by loading the skin, setting the title, and setting the content area to be empty.
modules/Demo/Controllers/HelloWorldController.php
<?php namespace SproutModules\Karmabunny\Demo\Controllers; use Sprout\Controllers\Controller; use Sprout\Helpers\View; class HelloWorldController extends Controller { public function index() { $view = new View('skin/inner'); $view->page_title = 'Hello World!'; $view->main_content = ''; echo $view->render(); } }
In order to access this page, we need a route. Once the route has been saved, the page will be accessible from http://your-server-name/hello-world
modules/Demo/config/routes.php
<?php $config['hello-world'] = 'SproutModules\\Karmabunny\\Demo\\Controllers\\HelloWorldController/index';
Next, there needs to be actual content on the page. This is achieved by loading an inner view and optionally populating it with data.
In the following updated example, the user is asked to enter their name. One they've submitted the form, they'll be greeted with today's date. The results should look like this:
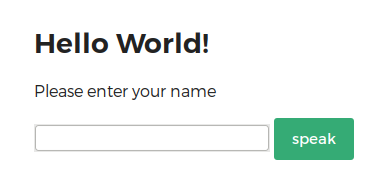
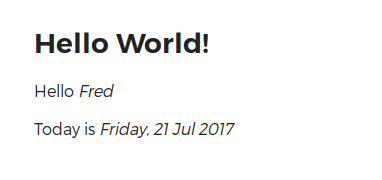
modules/Demo/Controllers/HelloWorldController.php
<?php namespace SproutModules\Karmabunny\Demo\Controllers; use Sprout\Controllers\Controller; use Sprout\Helpers\View; class HelloWorldController extends Controller { public function index() { if (empty($_GET['name'])) { $view = new View('modules/Demo/hello_form'); } else { $view = new View('modules/Demo/hello_message'); $view->name = $_GET['name']; } $skin = new View('skin/inner'); $skin->page_title = 'Hello World!'; $skin->main_content = $view; echo $skin->render(); } }
modules/Demo/views/hello_form.php
<form> <p><label for="name-field">Please enter your name</label></p> <p><input id="name-field" name="name" type="text"> <button type="submit" class="button button-green">Speak</button></p> </form>
modules/Demo/views/hello_message.php
<?php use Sprout\Helpers\Enc; ?> <p>Hello <em><?= Enc::html($name); ?></em></p> <p>Today is <em><?= Enc::html(date('l, j M Y')); ?></em></p>
While this example only has one method defined, any number of public methods can be added to the controller. Each will need a route in order to be accessible.
Regular expressions can be used in routes to capture patterns and convert them to function parameters.